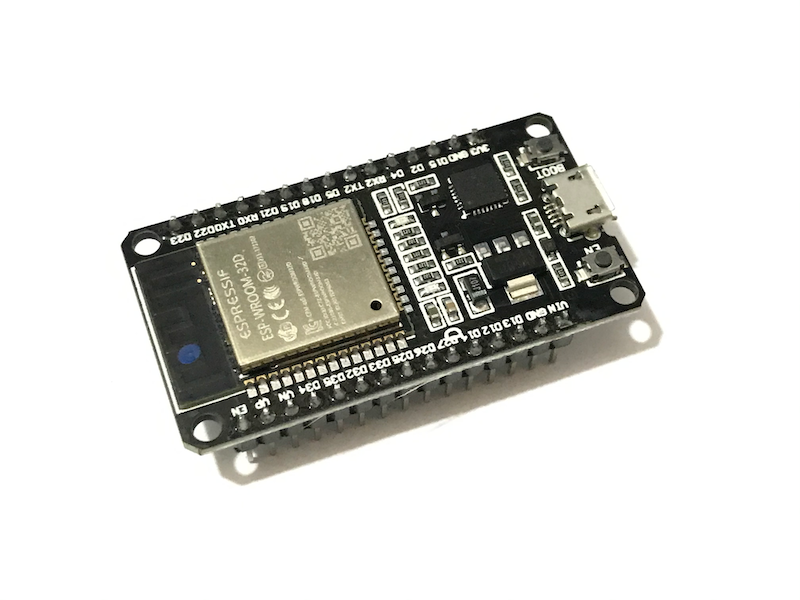
Introduction
ESP32 is an inexpensive microcontroller with Wifi and Bluetooth built-in. It is also Arduino compatiable, which means we can write programs for it with the Arduino IDE. While we love the powerful Arduino libraries, writing C++ codes can be messy in some situations, especially when we need to do rapid prototyping. Luckily, it is possible to use Python to write programs to control ESP32, thanks to the MicroPython support for ESP32.
MicroPython is an implementation of the Python programming language for microcontrollers. One of the most popular microcontrollers, the BBC micro:bit, can be programmed with MicroPython. On the other hand, Adafruit’s fork of MicroPython, CircuitPython, can also be run on a wide variety of microcontrollers or even a Raspberry Pi.
In this tutorial, we will install MicroPython on an ESP32 microcontroller, and write a simple blink program for the on-board LED.
A Few Words on the Computer
We will need Python and the Thonny IDE on the computer connecting to the ESP32. While you can use Linux, macOS or Windows, it’s recommended to use a Raspberry Pi computer, as the Raspbian OS comes with the drivers for many microcontrollers, and the Thonny IDE is pre-installed in Raspbian as well. If you do not use a Raspberry Pi computer, you need to download and install the Thonny IDE first. If you use Windows, make sure that Python has been installed and configured properly before you move on.
Install esptool
First, we need to install the esptool
program. This is a Python program that helps us flash software to ESP8266 and ESP32 microcontrollers.
In the terminal, use the pip3
command to upgrade or install the required Python packages:
pi@raspberrypi:~$ pip3 install --user --upgrade pip esptool
This will upgrade the pip
package and install the esptool
.
Flash the firmware
Connect the ESP32 to the computer. Make sure you use a micro-USB cable that can transfer data. Then, download the MicroPython firmware for ESP32 from the MicroPython website.
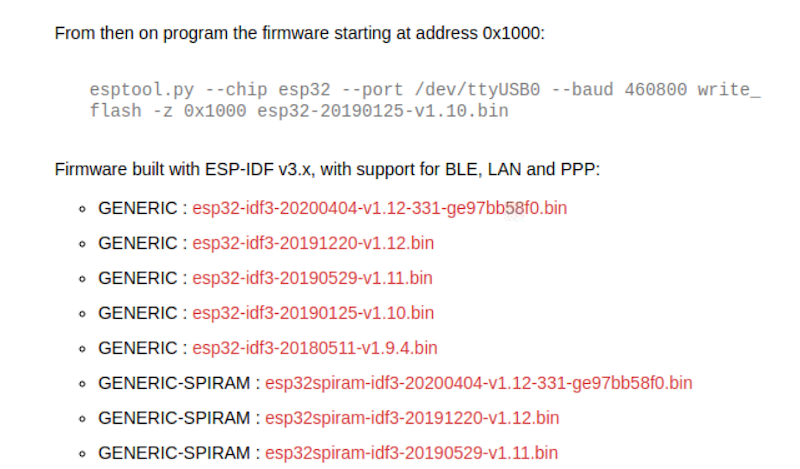
When the download is completed, launch the Thonny IDE.
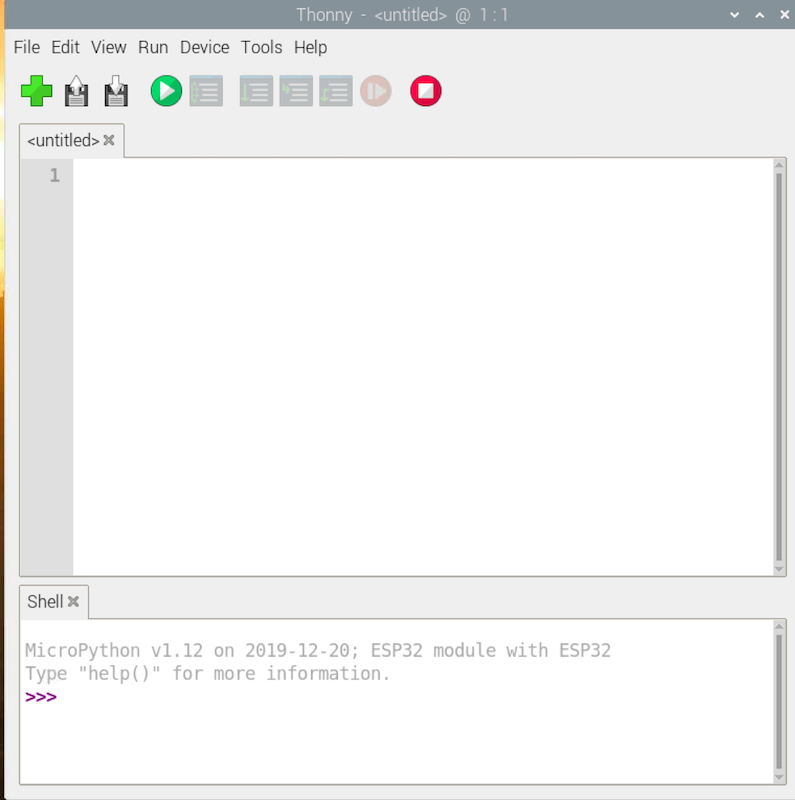
NOTE: Make sure the Thonny IDE is in ‘regular’ mode, NOT the ‘simple’ mode.
In the menu, click Tools → Options. Then, select the Interpreter tab. In ‘Which interpreter or device should Thonny use for running your code?’, select ‘MicroPython (ESP32)’. In ‘Port’, select the serial port connected to the ESP32 board.
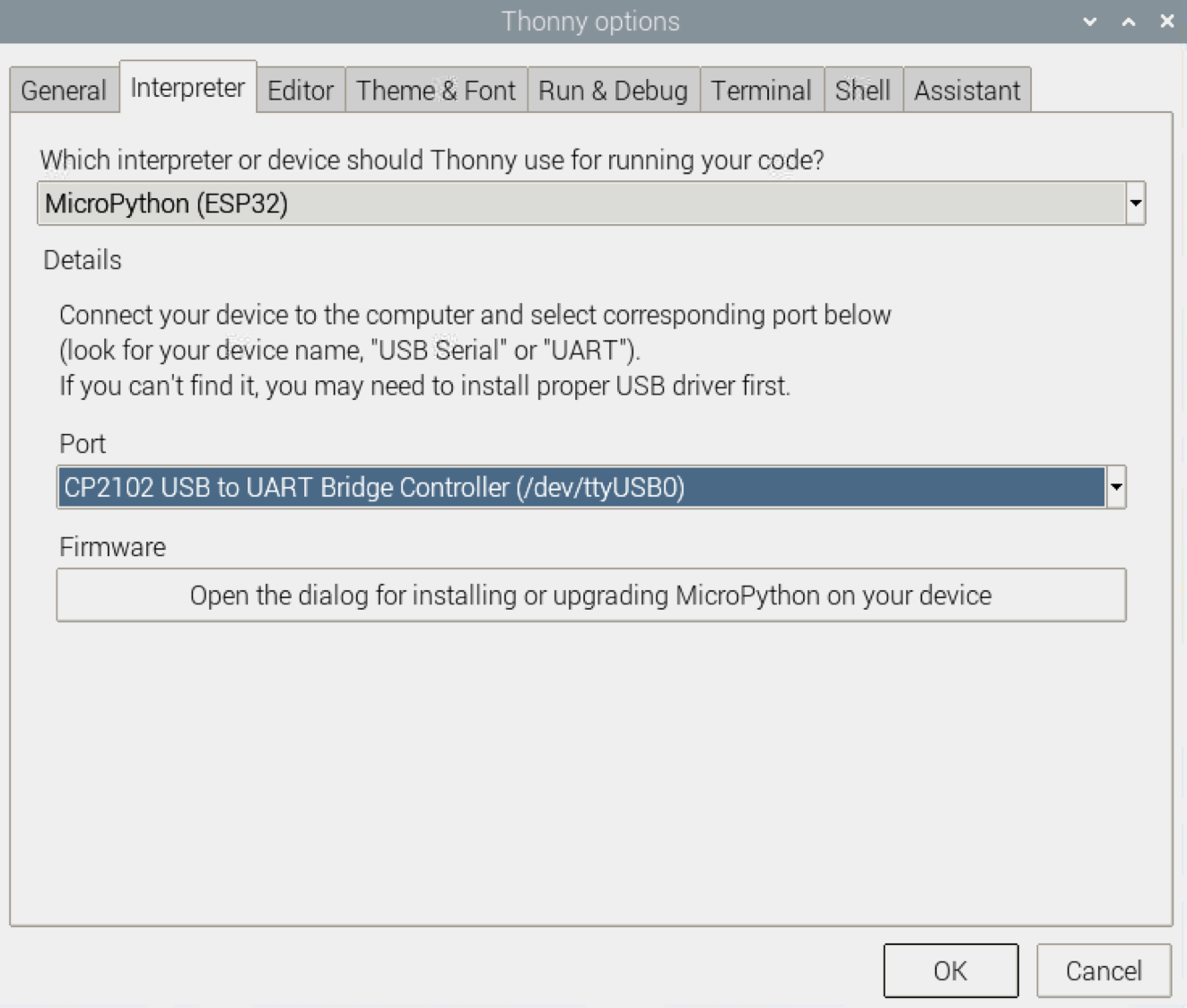
Then, click ‘Open the dialog for installing or upgrading MicroPython on your device’ under ‘Firmware’. A new window should appear. Select the serial port connected to the ESP32 board in ‘Port’, and select the firmware file we have downloaded. Make sure that ‘Erase flash before installing’ is selected.
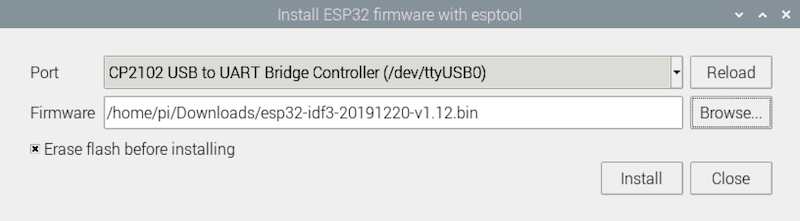
Finally, click ‘Install’ to flash the firmware to the ESP32.
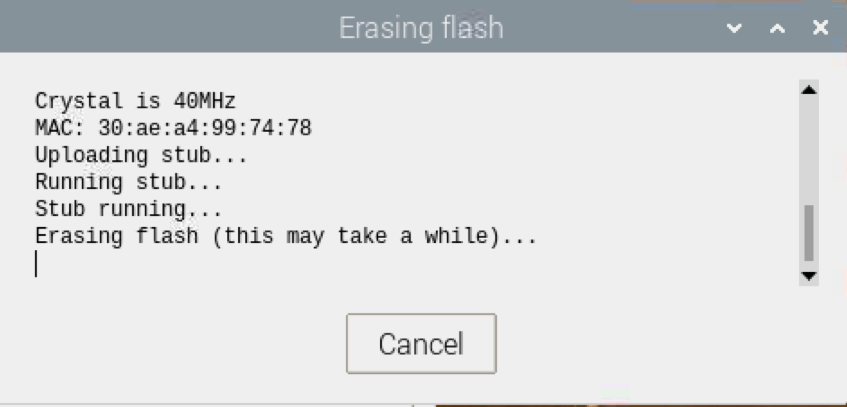
NOTE: For some ESP32 boards, you may need to push the ‘EN’ and the ‘BOOT’ buttons on the board to get the flashing started.
Use the REPL
When the firmware is installed to the ESP32, we can use the MicroPython’s REPL environment with the Thonny editor. First, click the red ‘Stop/Restart backend’ button to restart the MicroPython REPL.
NOTE: You can also use the shortcut key ‘Ctrl + D’ to initiate a soft-reboot of the ESP32 board.
At the bottom of the window, the shell can be used to interact with the REPL directly. Try typing a line of code inside the shell:
print("Hello World")
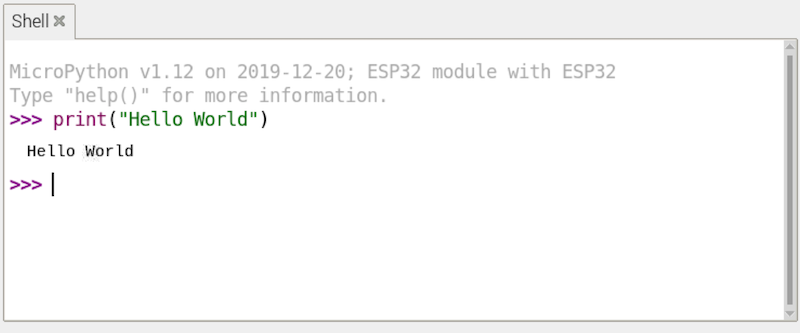
However, the codes inside the REPL are gone everytime the REPL is restarted. So a better way to write our codes is to write them inside the editor (obviously!). Let’s write a simple program to flash the onboard LED 10 times.
from machine import Pin
from time import sleep
def flash(led, num_of_times):
for i in range(num_of_times):
led.on()
print('ON')
sleep(1)
led.off()
print('OFF')
sleep(1)
led = Pin(2, Pin.OUT)
flash(led, 10)
You may save the program to your computer as usual. When you encounter the following dialog box, you can just choose ‘This Computer’.
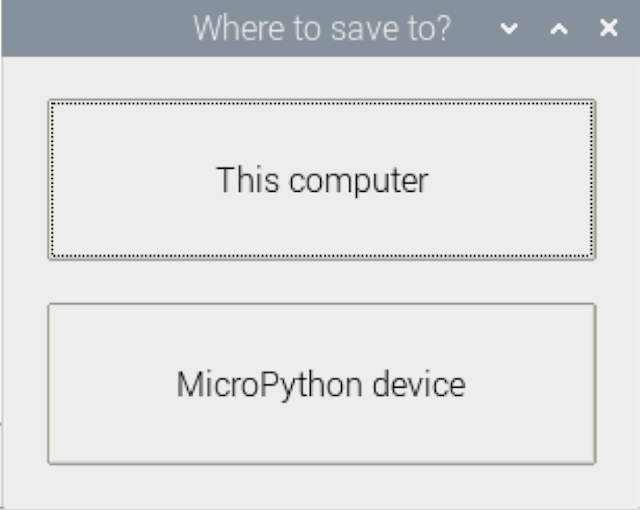
Click the red ‘Stop/Restart backend’ button to restart the MicroPython REPL to stop the previous running program, if any. Then, click the green ‘Run current script’ button to run the script.
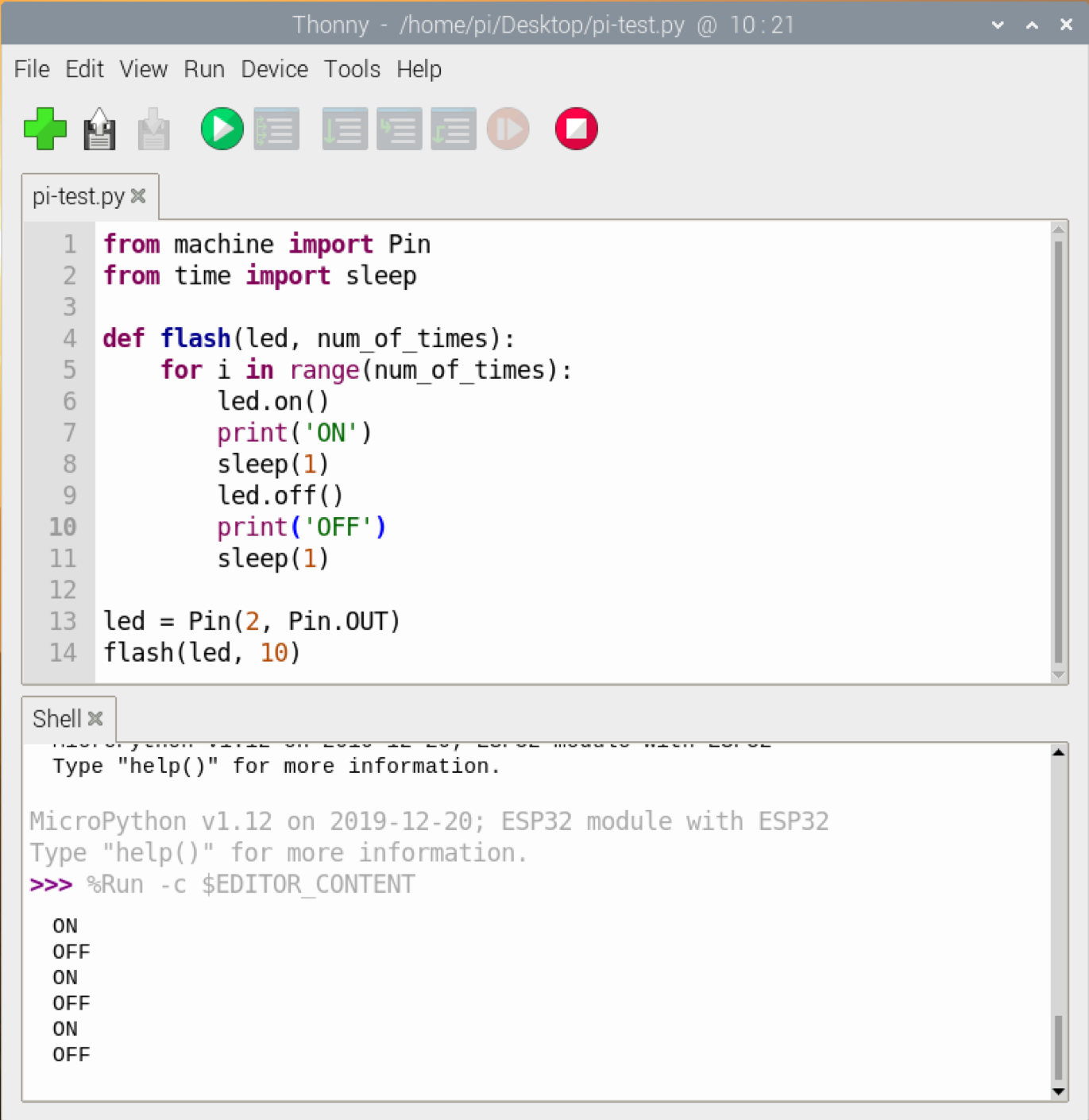
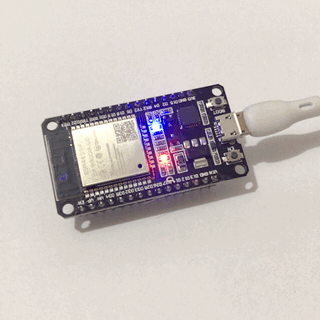
Save Codes to the Board
As suggested by the dialog box shown when we save a file, we can actually save a file to the ESP32 directly. When a Python file is saved to the ESP32, it can be imported as a Python library as usual. Also, if the Python file is named ‘main.py’, the codes inside the file will be executed automatically when the board is powered up. That is to say, ‘main.py’ is like the program we upload to an Arduino board.
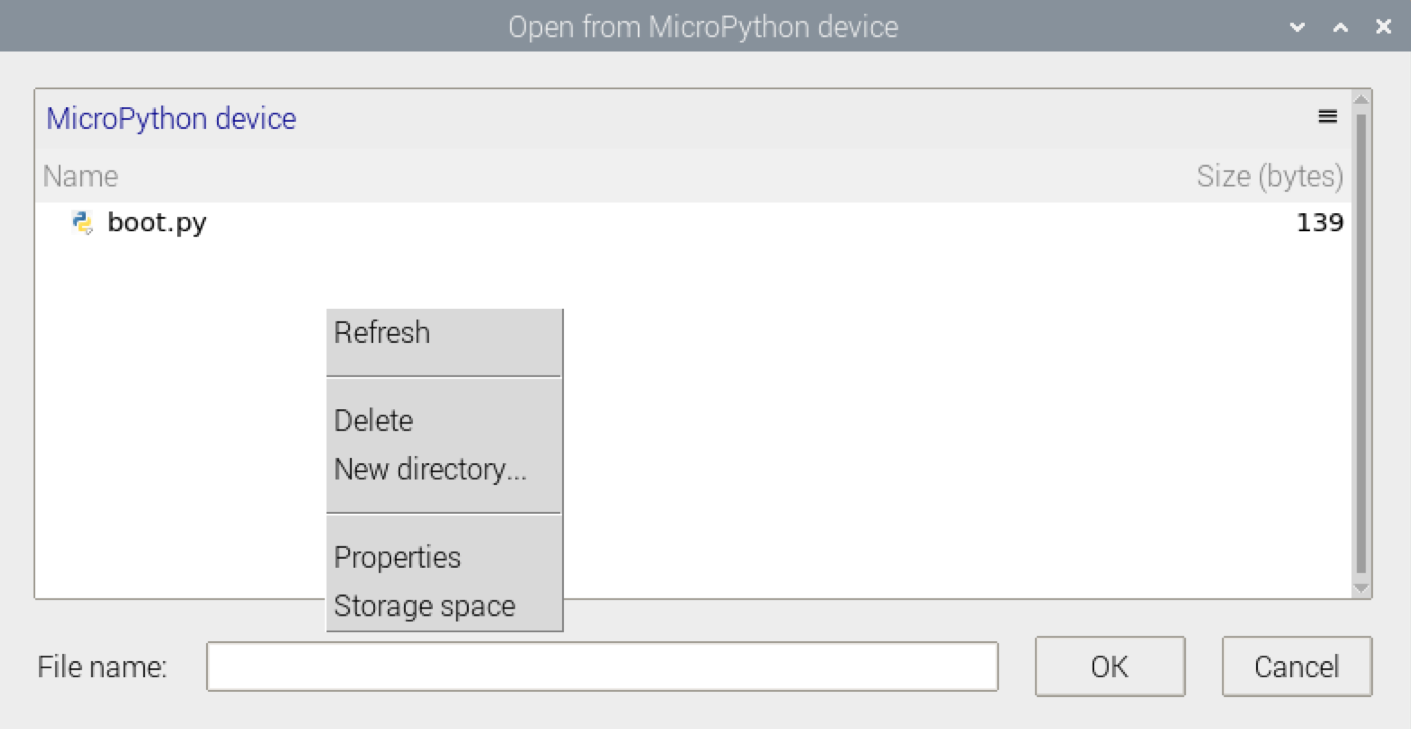
Conclusion
With this simple setup, we can use MicroPython to write programs for ESP32. Other than controlling the GPIOs, we can use MicroPython to connect to the Internet. Various MicroPython libraries allow us to send HTTP requests, start a web server or even perform co-operative multitasking. Though the performance of MicroPython is not as good as C++, in many cases this is not an issue. Since the codes can just run inside REPL, the debugging process is a lot easier. The combination of MicroPython and ESP32 enables us to do rapid prototyping for IoT devices.